π Unleash
Unleash is a robust feature flagging solution that you can roll out to your team easily today. It's easy to use, comes with a lot of features and support for both server-side and client-side languages and frameworks.

Great software teams feature flag. Unleash (code) provides a F/OSS off-the-shelf solution to feature flagging that you can bring to your team today. Here's how it's architected:
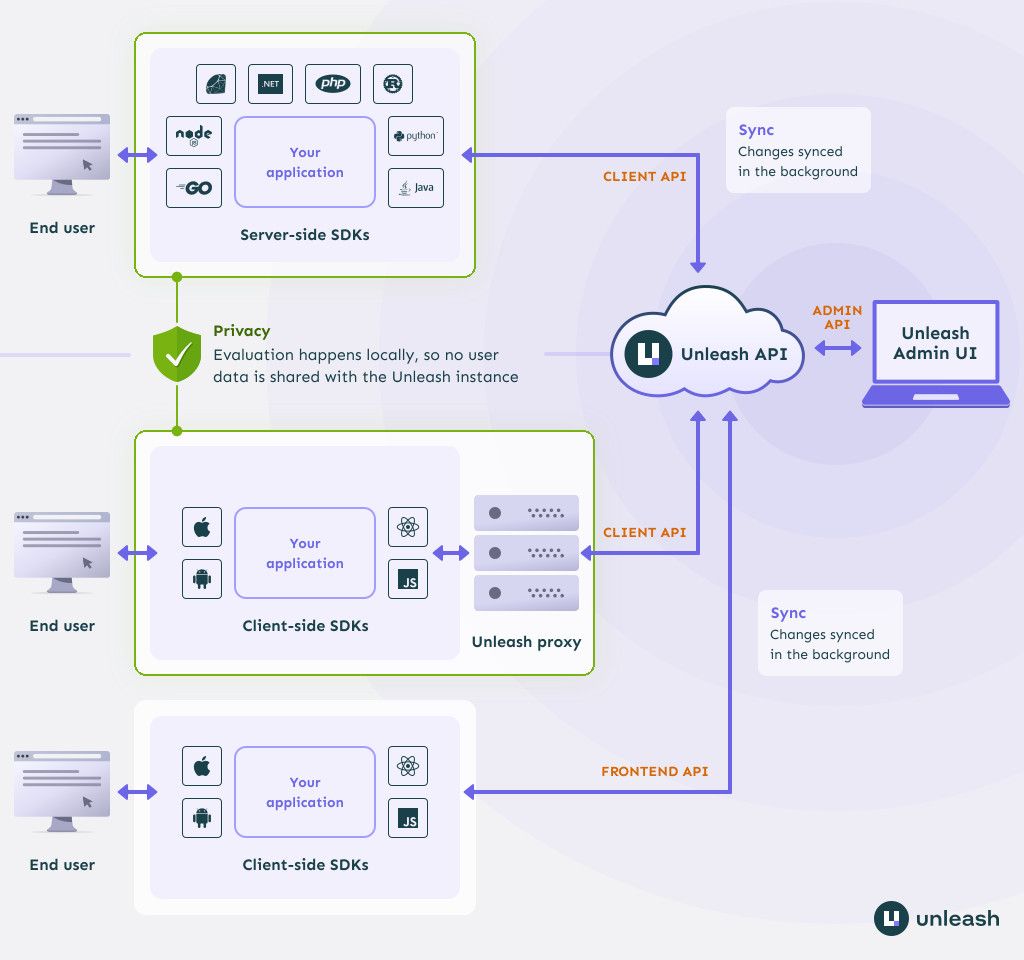
The diagram is a little busy, but the server-side usage is a bit clearer:
// Unleash server side usage (NodeJS)
const { initialize } = require('unleash-client');
const unleash = initialize({
url: 'https://YOUR-API-URL',
appName: 'my-node-name',
customHeaders: { Authorization: 'SOME-SECRET' },
});
// Usage of a specific toggle
const demoToggle = unleash.isEnabled('DemoToggle');
And in various clients (web apps, mobile apps, etc):
// Unleash client side usage (iOS w/ Swift)
import SwiftUI
import UnleashProxyClientSwift
// Setup Unleash in the context where it makes most sense
var unleash = UnleashProxyClientSwift.UnleashClient(unleashUrl: "https://<unleash-instance>/api/frontend", clientKey: "<client-side-api-token>", refreshInterval: 15, appName: "test")
unleash.start()
While in the past teams may have used environment variables or home-grown configuration sources (Database, files, APIs) to see whether a feature toggle is available, Unleash provides a solution that is easy to roll out and easy to manage (that your team doesn't have to maintain!).
Unleash can be controlled and driven via API (it also has a OpenAPI/Swagger spec!), so it can be integrated into many workflows quite easily, here's a curl
example:
curl -H "Content-Type: application/json" \
-H "Authorization: MY-ADMIN-API-KEY" \
-X POST \
-d '{
"name": "my-unique-feature-name",
"description": "lorem ipsum..",
"type": "release",
"enabled": false,
"stale": false,
"strategies": [
{
"name": "default",
"parameters": {}
}
],
"variants": [],
"tags": []
}' \
http://unleash.yoursite.tld/api/admin/features
Unleash has extensive documentation and very clear guides on how it's built and how to do various kinds of testing, like A/B testing.
Running Unleash
Unleash is quite docker
friendly, with support for both running docker-compose
and docker
to start the individual pieces.
You can use the Unleash docker-compose.yml
, which looks like this:
version: "3.9"
services:
# The Unleash server contains the Unleash configuration and
# communicates with server-side SDKs and the Unleash Proxy
web:
image: unleashorg/unleash-server:latest
ports:
- "4242:4242"
environment:
# This points Unleash to its backing database (defined in the `db` section below)
DATABASE_URL: "postgres://postgres:unleash@db/postgres"
# Disable SSL for database connections. @chriswk: why do we do this?
DATABASE_SSL: "false"
# Changing log levels:
LOG_LEVEL: "warn"
# Proxy clients must use one of these keys to connect to the
# Proxy. To add more keys, separate them with a comma (`key1,key2`).
INIT_FRONTEND_API_TOKENS: "default:development.unleash-insecure-frontend-api-token"
# Initialize Unleash with a default set of client API tokens. To
# initialize Unleash with multiple tokens, separate them with a
# comma (`token1,token2`).
INIT_CLIENT_API_TOKENS: "default:development.unleash-insecure-api-token"
depends_on:
db:
condition: service_healthy
command: [ "node", "index.js" ]
healthcheck:
test: wget --no-verbose --tries=1 --spider http://localhost:4242/health || exit 1
interval: 1s
timeout: 1m
retries: 5
start_period: 15s
db:
expose:
- "5432"
image: postgres:15
environment:
# create a database called `db`
POSTGRES_DB: "db"
# trust incoming connections blindly (DON'T DO THIS IN PRODUCTION!)
POSTGRES_HOST_AUTH_METHOD: "trust"
healthcheck:
test:
[
"CMD",
"pg_isready",
"--username=postgres",
"--host=127.0.0.1",
"--port=5432"
]
interval: 2s
timeout: 1m
retries: 5
start_period: 10s
Check out the documentation on how to get started for more information.
For greater control, you can also start the official container image manually:
docker run \
-p 4242:4242 \
-e DATABASE_HOST=postgres \
-e DATABASE_NAME=unleash \
-e DATABASE_USERNAME=unleash_user \
-e DATABASE_PASSWORD=some_password \
-e DATABASE_SSL=false \
--name unleash \
--pull=always unleashorg/unleash-server
The official docker image has instructions and is well-maintained as well.
Note that the above docker run
command assumes that you have a Postgres database Β running, so you might want to start that first (and read up on how to use/maintain the database!):
docker run \
-e POSTGRES_USER=unleash_user \
-e POSTGRES_PASSWORD=some_password \
-e POSTGRES_DB=unleash \
--name postgres \
postgres