⭐ThreeJS
If you encounter any issues (or if you prefer), just send an email with the subject "unsubscribe" to admin@awsmfoss.com – I'll unsubscribe you myself.
Three.js is a JavaScript library for easily creating 3D graphics on the web, utilizing WebGL for seamless integration of immersive visuals into websites and applications.
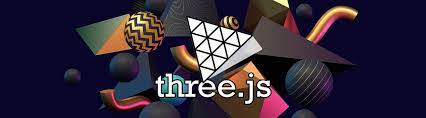
Three.js (Code) is an open-source JavaScript library designed to simplify the creation and rendering of 3D graphics on the web.
Built on top of the WebGL API, Three.js provides a high-level abstraction, making it accessible for developers to incorporate 3D models, animations, and interactive elements into their websites or applications with ease.
ThreeJS offers a comprehensive set of features, including scene management, camera controls, lighting, materials, and geometry, enabling the development of immersive and visually appealing online experiences. With a large and active community, Three.js continues to evolve, making it a versatile and widely adopted tool for web-based 3D graphics development.
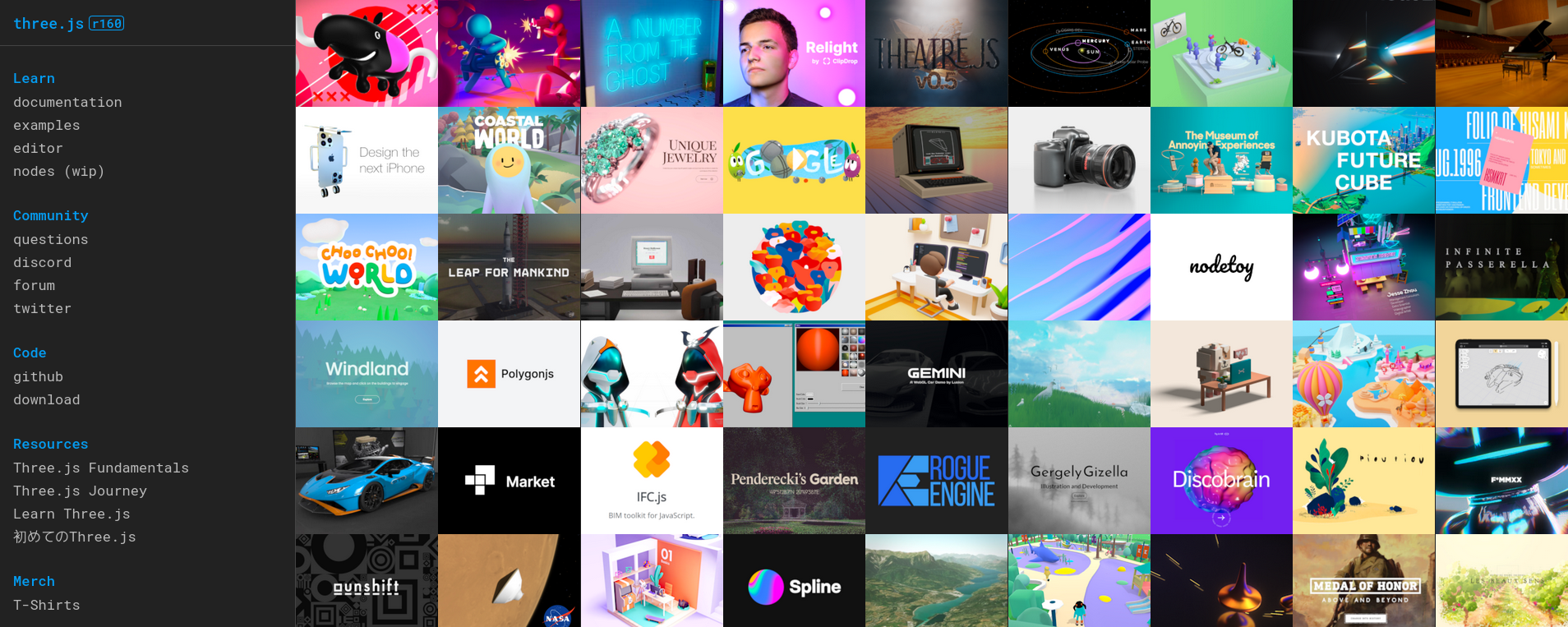
Check out the things people are building with ThreeJS on the Examples page:
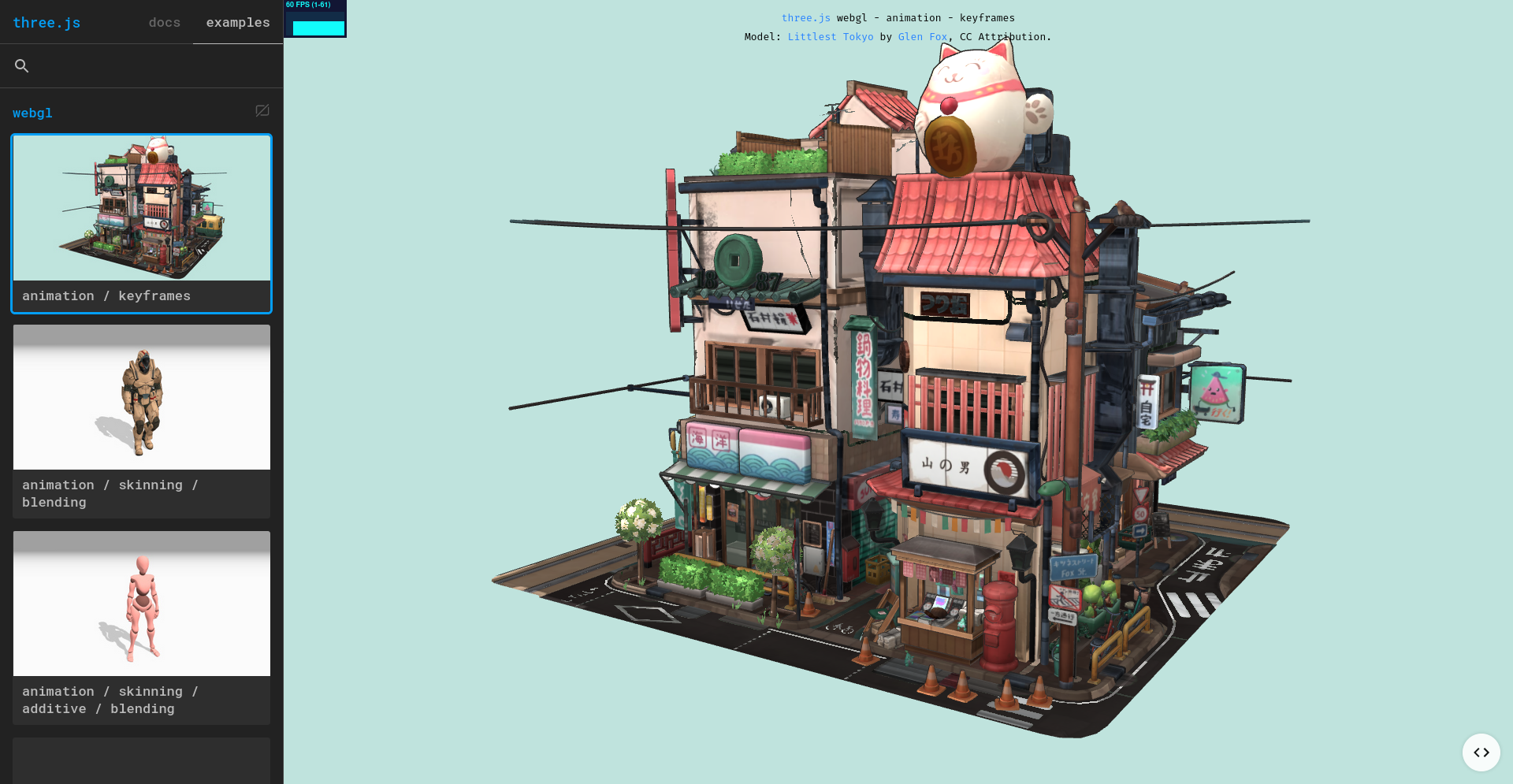
🤖 As described by AI
Three.js is a JavaScript library that simplifies the creation of 3D graphics on the web, offering a user-friendly interface over WebGL. With a vibrant community, extensive documentation, and support for VR/AR, it enables developers to easily integrate immersive 3D experiences into web applications.
✅ Features
ThreeJS has a lot of features that enable 3D development:
- WebGL Abstraction: Simplifies WebGL complexities for accessible 3D content creation.
- Scene Management: Organizes 3D elements within scenes for effective environment structuring.
- Cameras and Controls: Supports various cameras and user interaction controls for scene navigation.
- Geometry and Materials: Provides predefined shapes and materials for easy 3D object creation.
- Lights and Shadows: Offers diverse lighting options and shadow implementation for realistic scenes.
- Animation: Enables smooth animations with keyframes and dynamic effects.
- Post-processing Effects: Includes effects like bloom and motion blur for enhanced visuals.
- Raycasting: Supports raycasting for mouse picking and interaction scenarios.
- Exporters and Importers: Allow importing external 3D models and exporting scenes in incompatible formats.
- Cross-browser Compatibility: Ensures consistent 3D experiences across different web browsers.
- Responsive Design: Adapts to various screen sizes, making it suitable for desktop and mobile devices.
with ThreeJS we can animate our site more efficiently and more attractive.
👟 Getting started with ThreeJS
Setup a Local Development Environment:
# Example for installing Node.js and npm (Linux)
sudo apt-get update
sudo apt-get install nodejs
sudo apt-get install npm
- Install Node.js and npm: https://nodejs.org/
Create a Basic HTML File:
- Create an HTML file using a text editor.
touch index.html
code index.html # Opens Visual Studio Code with the newly created file
Include Three.js Library:
- Download Three.js or use a CDN.bash
# Download Three.js (if needed)
wget https://threejs.org/build/three.min.js
Set Up a Scene:
- Open
index.html
and add the following code.html
<script>
// Your Three.js code goes here
</script>
Create a Scene, Camera, and Renderer:
- Add the following code to the script tag.
var scene = new THREE.Scene();
var camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
var renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
Add a Cube:
- Continue adding the cube code. javascript
var geometry = new THREE.BoxGeometry();
var material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
var cube = new THREE.Mesh(geometry, material);
scene.add(cube);
Animate the Cube:
- Add the animation code. javascript
function animate() {
requestAnimationFrame(animate);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
Run Your Local Server:
- Install and start a local server.
npm install -g http-server
http-server
Open Your Browser:
- Open a web browser and navigate to
http://localhost:8080
or the address provided by your local server.
sudo apt-get update
sudo apt-get install docker-ce docker-ce-cli containerd.io
Inspect and Debug:
- Use browser developer tools to inspect and debug your Three.js application.
See more details on the ThreeJS Getting Started page.