⚡ neo4j-php/Bolt
Bolt is a Neo4j driver written for the always-more-popular-than-you-expect PHP programming language.
Before you can understand what Bolt is you probably want to understand Neo4j – it's a graph database engine, which means it makes it easy to use data that is connected by relationships (more so than simply tabular data).
Here's a quick intro to Neo4j (the whole playlist is great):
If you're writing code for PHP, and you want to connect to a Neo4j database, then bolt
is one of the best ways to do that.
🌠 Features
Usage of bolt
requires a bit of work on the client/development side but it is chock full of features:
composer
powered installation is available (it's listed)- Concise & informative documentation/README
- Supports SSL & self signed certs
- Supports PDO via an add-on called
pdo-bolt
- Used in
neo4j-php-client
- Support for PHP 7, 8 and newer
🤖 As described by AI
The project "neo4j-php/Bolt" is a PHP library designed to enable connectivity to graph databases over TCP socket using the Bolt protocol, which is specified by Neo4j. It aims to be low-level, supporting all available versions of the Bolt protocol, and keeps up with the protocol's architecture and specifications.
The library supports Bolt protocol versions up to 5.4 and requires PHP version 8.1 or higher, along with specific PHP extensions for full functionality. It can be installed via composer or manually, and it provides a comprehensive set of methods for interacting with a Neo4j database, including authentication, transaction management, and query execution. For more details, you can visit the project's GitHub page: [neo4j-php/Bolt](https://github.com/neo4j-php/Bolt).
📺 Watch this
If you wanted to get started with PHP & Neo4j but don't want to read about it, we have the playlist for you:
👟 Getting started
To get started locally, you'll need a PHP application and a Neo4j database to work with.
Starting Neo4j locally
To start Neo4j locally, you can use the official neo4j
docker image:
docker run \
--publish=7474:7474 \
--publish=7687:7687 \
--volume=$HOME/neo4j/data:/data \
neo4j
As you might expect, the docker
command above does a few things:
- Publishes Neo4j HTTP access port
7474
in the container to your machine (127.0.0.1:7474
and0.0.0.0:7474
) - Publishes Neo4j Bolt access port
7687
in the container to your machine (127.0.0.1:7687
and0.0.0.0:7687
) - Mounts and uses
~/neo4j/data
as the container-internal data directory (so your data will survive the container restarting)
Of course, since we're using bolt
for PHP, we can enable only the bolt access port if we want.
Integrating bolt
into your PHP app
While we can't guide you on how to actually build a good PHP app (well we could, but it would take a long time), we can at least note the integration steps.
Since bolt
offers a client, you generally build a client so you can connect to the Neo4j server that is running:
// Choose and create connection class and specify target host and port.
$conn = new \Bolt\connection\Socket('127.0.0.1', 7687);
// Create new Bolt instance and provide connection object.
$bolt = new \Bolt\Bolt($conn);
// Set requested protocol versions ..you can add up to 4 versions
$bolt->setProtocolVersions(5.4);
// Build and get protocol version instance which creates connection and executes handshake.
$protocol = $bolt->build();
Once you have a client connection (that $conn
object), you can perform initialization:
// Initialize communication with database
$response = $protocol->hello()->getResponse();
As noted in the README
, you should verify that the results of $response
there are successful – it's possible that the hello
handshake fails.
Once you're confirmed to be connected, you can authenticate yourself (if auth is set up):
// Login into database
$response = $protocol->logon(['scheme' => 'basic', 'principal' => 'neo4j', 'credentials' => 'neo4j'])->getResponse();
And start to send messages:
// Pipeline two messages. One to execute query with parameters and second to pull records.
$protocol
->run('RETURN $a AS num, $b AS str', ['a' => 123, 'b' => 'text'])
->pull();
// Fetch waiting server responses for pipelined messages.
foreach ($protocol->getResponses() as $response) {
// $response is instance of \Bolt\protocol\Response.
// First response is SUCCESS message for RUN message.
// Second response is RECORD message for PULL message.
// Third response is SUCCESS message for PULL message.
}
All of this is in the README, and while the PHP driver isn't yet listed (as of now) on the official Bolt drivers listing, you can check out the protocol if you're interested in the low level details:
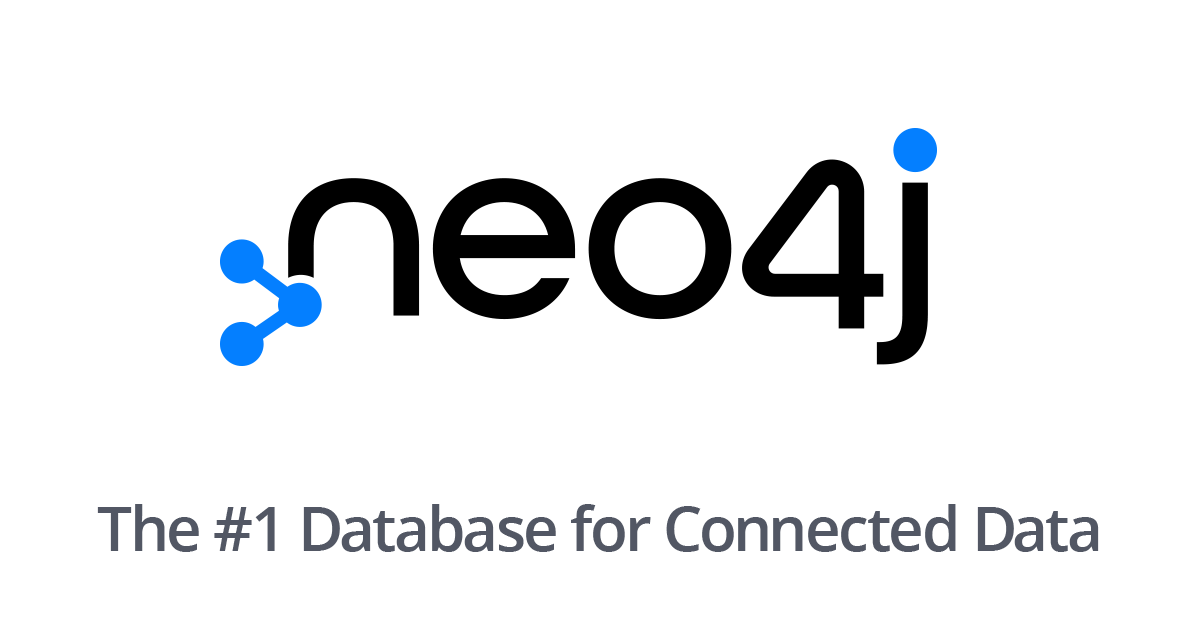
🧑💻 Want to contribute?
If you find any bugs or want to improve the codebase, bolt
is open to new issues:
There are over 5 contributors and over 100 users on GitHub so there's definitely an ecosystem to depend on there as you submit improvements/fixes, or test things out.